Bluetooth Testing with Smartphone App
Hardware + Software Requirements
You will need an Arduino with Bluetooth capability to follow this tutorial. (We have used the Bluno and the Bluno Beetle.) You will also need to download Arduino's open source IDE.
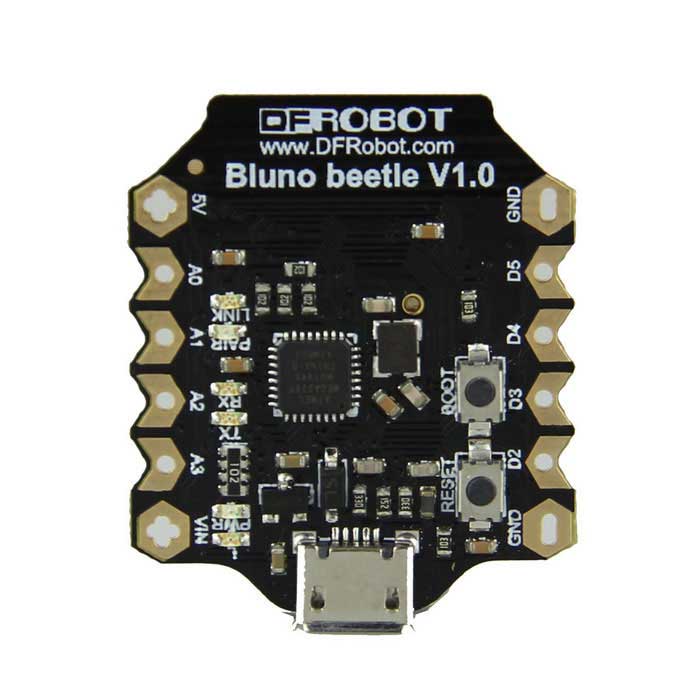
If you have an Android phone, then you will need to install Apache Cordova software onto your computer in order to deploy the app on your phone.
If you are using an iPhone, you will need to download Evothings Viewer from the App Store to deploy the app.
Arduino (Bluno Version) Code
Below is the code you can copy into your Arduino project.
// Arduino code for Aerotenna's uLanding app.
int altitude = 0;
// This function is called only once, at reset.
void setup() {
Serial.begin(115200);
Serial.println("Hello world!");
delay(2);
}
// This function is called continuously, after setup() completes.
void loop() {
byte temp_alt = 0;
byte temp_alt_2 = 0;
byte first_byte = 0;
unsigned long past_time = micros();
unsigned long cur_time = micros();
//Wait for first byte, just in case it thinks the second or third byte is
//the first.
while (cur_time - past_time < 400) {
if (Serial.available()) {
cur_time = micros();
}
else {
cur_time = micros();
past_time = cur_time;
Serial.flush();
}
}
//wait for all bytes to be available
while (Serial.available() < 3);
if (Serial.available()) {
first_byte = Serial.read();
first_byte &= 0x7F;
}
if (Serial.available()) {
temp_alt = Serial.read();
temp_alt &= 0x7F;
}
if (Serial.available()) {
temp_alt_2 = Serial.read();
temp_alt_2 &= 0x7F;
}
altitude = temp_alt | (temp_alt_2 << 7);
byte buffer[3] = {0xAD,
altitude,
(byte)(altitude >> 8)
};
Serial.write(buffer, sizeof(buffer));
}
Connect Arduino to μLanding
The next step is to connect Arduino to μLanding. You will need to supply 5V power to both the Arduino and μLanding. We have done this using a USB-chargeable battery for mobility.
- Connect POWER to the VIN pin on Arduino, and the RED wire on μLanding (unless you plan to power the Arduino using USB).
- Connect GND to the GND pin on Arduino, and the BLACK wire on μLanding.
- Connect digital pin RX on Arduino to the ORANGE wire on μLanding.
Smartphone App
Download the app project files from GitHub here.
You can also get the source files using git, with the following command:
$ git clone https://github.com/Aerotenna/uLanding_app.git
Follow the appropriate instructions below, whether you are using Android or iOS.
Android
Open a terminal in Windows (or Mac) and navigate to the Cordova Projects directory. Run the following commands:
$ cordova create uLanding com.Aerotenna.uLanding uLanding
$ cd uLanding
Replace everything in the www folder of the project you created with everything in the www folder of the project you downloaded from us. You will need to add a few plugins to your project to enable Bluetooth communications.
$ cordova plugin add cordova-plugin-ble
$ cordova plugin add cordova-plugin-ble-central
$ cordova plugin add cordova-plugin-bluetooth-serial
$ cordova plugin add cordova-plugin-estimote
$ cordova plugin add cordova-plugin-whitelist
You will need to add the platform for an Android device:
$ cordova platform add android
Next, build the project:
$ cordova build android
Then you will need to enable debugging on your Android device. Follow these instructions to do that.
Finally, plug in your phone to your computer via a micro USB to USB cable, and then in your Cordova project in a terminal, type:
$ cordova run android
It should detect your phone and deploy the app.
iPhone
Open up EvoThings on your desktop and follow the instructions to activate the software. Then you will need to connect your device to your desktop using the provided key. Follow the simple instructions in EvoThings to get connected.
Click the Examples tab at the top menu to see a list of example projects. Scroll down until you see Bluno - Hello World, and click COPY to create a copy of it. Name the project uLanding and then click CREATE.
Now click MY APPS and you should see the project you just copied.
This is the important step. Replace everything in that project directory with everything from the www folder within the project you downloaded from us.
Finally once your phone is connected to EvoThings, you can press RUN and it should automatically download to your phone and you can now run the app.
Start and Play
Power up μLanding and the Arduino, and then run the app and press SCAN. It should find your Arduino Bluetooth device automatically. Click on the correct device, and it should start displaying the altitude information.
Updated less than a minute ago